INDEX
- Flask RESTPlus CRUD Board 만들기 실행 (1)
- Flask RESTPlus CRUD Board 만들기 설정 및 MySQL (2)
- Flask RESTPlus CRUD Board 만들기 ORM 그리고 Model (3)
- Flask RESTPlus CRUD Board 만들기 MVC 그리고 REST API Swagger (4)
- Flask RESTPlus CRUD Board 만들기 Auth 그리고 암호화 (5)
이번 포스트는 macOS Mojave 10.14.5 에서 수행된 작업입니다.
개발 환경
- MacBook Pro (13-inch, 2017, Four Thunderbolt 3 Ports)
- Python 3.7
- vscode
- Docker-Compose version 1.23.2, build 1110ad01
Authorization 붙이기 (PyJwt), Password 암호화
먼저, 클라이언트 측에서 회원가입을 할 때, Password
를 Database
에 저장 때는 암호화
해서 넣어야 합니다(개발자가 사용자 비밀번호 까지 알아서 뭐 할려고?)
그러기 위해서는 Bcrypt
를 사용해 암호화를 진행 할 것이며, 암호화 한 것을 Database
에 저장하고, 사용자가 로그인 할 때, 서버에서 발급해주는 Token
을 이용해 API를 사용할 것 입니다 Token
이 없다면 API
의 요청은 거절
하도록 만들 것 입니다
해봅시다!
PyJwt
종속성을 설치합니다
1 | > pipenv install PyJwt |
데코레이터
를 이용해서 구현한 API
에 붙여 주도록 하곘습니다
1 | # ./app/api/auth_type.py |
REST API Documents
에 Authorization
을 보여주기 위해 위와 같은 코드를 작성하고,Token 발급
을 위해 다음과 같이 코드를 작성하겠습니다
1 | # ./app/api/__init__.py |
1 | # ./app/users/views.py |
발급한 Token
을 클라이언트 측에서 저장을 한 뒤 발급받은 토큰을 서버로 Header
부분에 담아 데이터를 전송하여 맞으면 API요청
을 받는 코드를 작성하도록 하겠습니다
1 | # /app/posts/views.py |
Post Class
의 post함수
를 보게 되면 데코레이터
로 confirm_token
을 붙인 것을 볼 수 있습니다 이 API
로 요청이 왔을 때 먼저 토큰이 있는지 검사하고 통과 되면 Post함수
를 실행하게 되는 구조입니다
실행을 해봅시다
1 | $ python manage.py run |
아래와 같은 화면을 볼 수 있으며 각각 실행을 해보세요!
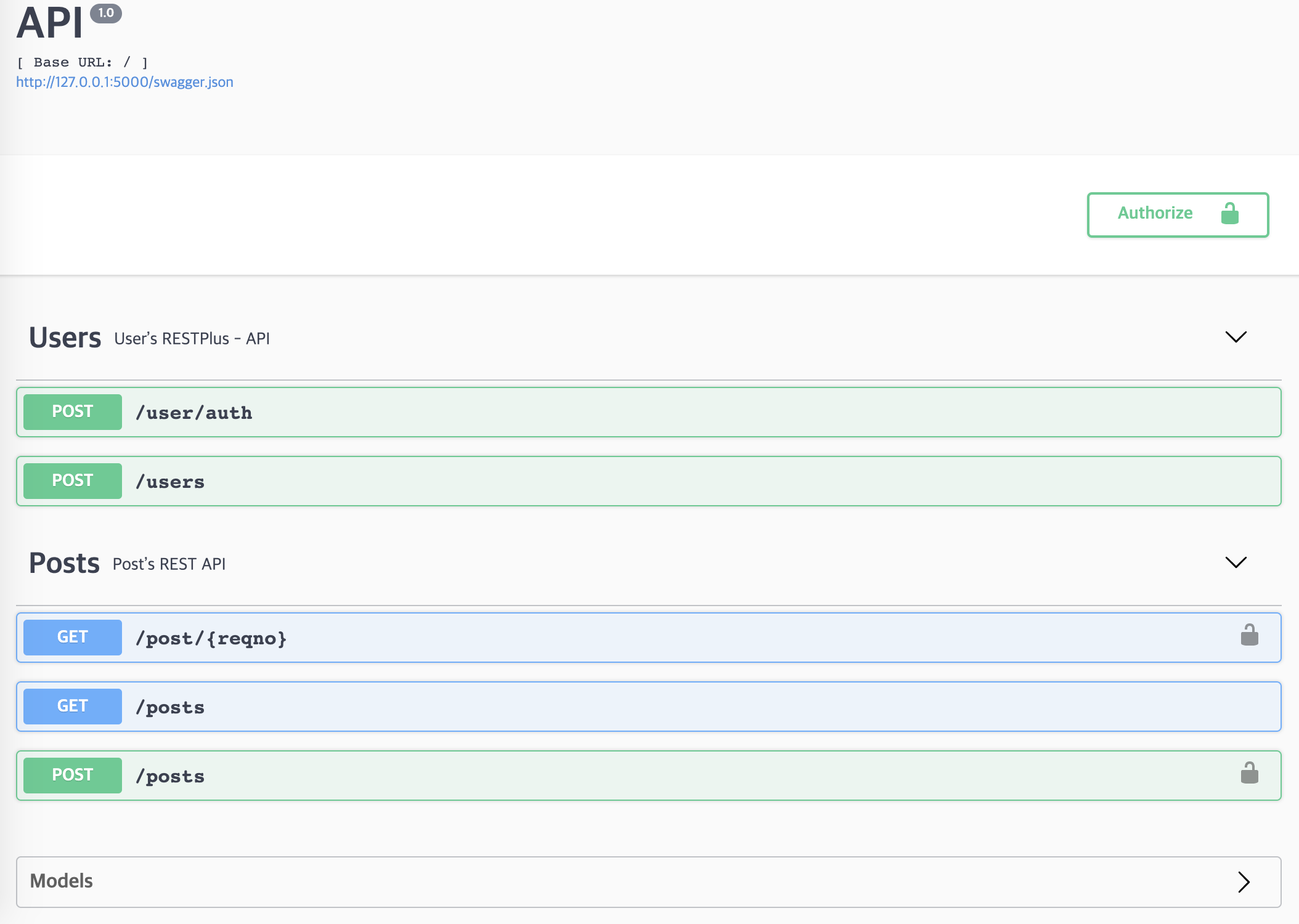
최종적인 폴더 구조는 다음과 같습니다
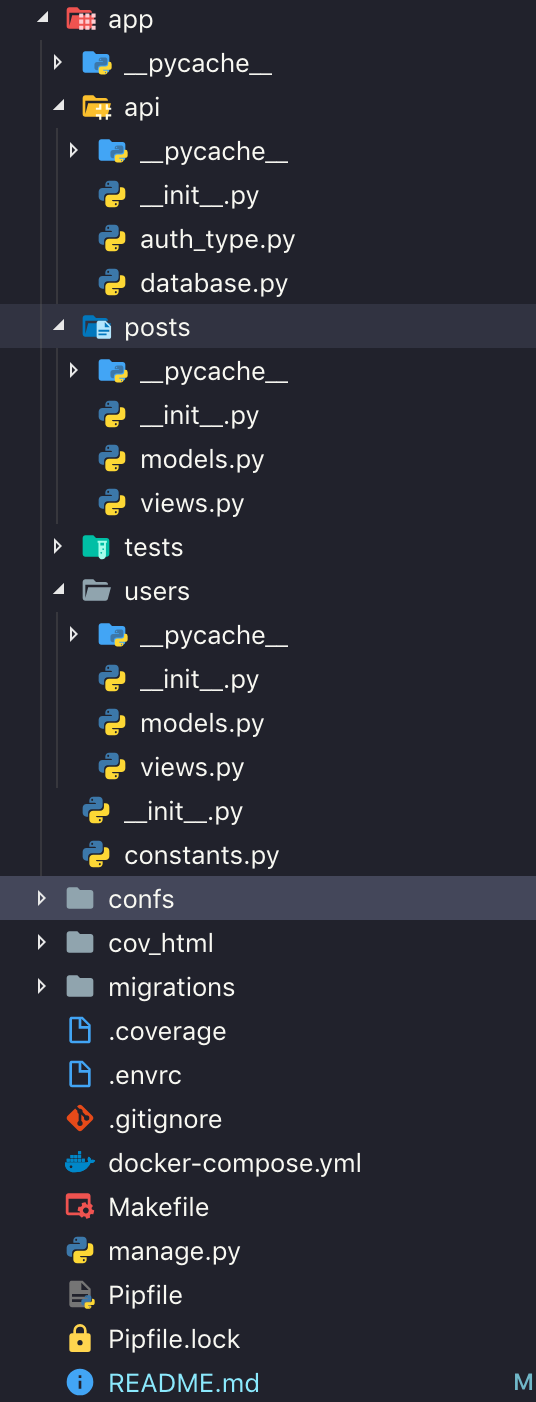
고생하셨습니다! 지금까지 따라와주셔서 정말 감사드리며 여기까지 하게 되면 Flask
프레임워크의
기본을 할 수 있게 된 것 입니다!